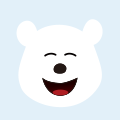
在工作中如何使用枚举(enum) 原创
春节不停更,此文正在参加「星光计划-春节更帖活动」
日积月累,水滴石穿 😄
什么是枚举
枚举是JDK 1.5
中引入的新特性,由一组固定的常量组成合法值的类型,例如一年中的季节、一周的星期数。枚举其实就是特殊的类,继承了java.lang.Enum
类,并实现了java.lang.Seriablizable
和 java.lang.Comparable
两个接口。域成员均为常量,且构造方法被默认为私有。
如何定义枚举
先来看看枚举是如何定义的!我们定义四个值,分别为 春天、夏天、秋天、冬天。
public enum SeasonEnum {
//春天
SPRING,
//夏天
SUMMER,
//秋天
AUTUMN,
//冬天
WINTER;
}
以上方式就是枚举类的定义方式。很简单!那我们再来看看枚举类提供的函数。
枚举的函数
public static void main(String[] args) {
//1、根据传入的名称获得指定的枚举,可能会抛出异常
SeasonEnum autumn = SeasonEnum.valueOf("AUTUMN");
System.out.println("autumn1 = " + autumn);
//与 1、一致
SeasonEnum anEnum = SeasonEnum.valueOf(SeasonEnum.class, "AUTUMN");
System.out.println("autumn2 = " +anEnum);
//2、返回当前枚举类中的所有元素
SeasonEnum[] values = SeasonEnum.values();
System.out.println("values = " +Arrays.toString(values));
//3、获得枚举元素
SeasonEnum name1 = SeasonEnum.AUTUMN;
System.out.println("name1 = " + name1);
//获得枚举元素的名称
String name2 = SeasonEnum.AUTUMN.name();
System.out.println("name2 = " + name2);
//4、返回此枚举元素的索引,从 0 开始
int ordinal = SeasonEnum.AUTUMN.ordinal();
System.out.println("ordinal = " + ordinal);
//5、与指定的对象进行比较,返回一个负整数,零或正整数。
//小于指定对象返回 负整数
//等于指定对象返回 零
//大于指定对象 返回正整数
int i = SeasonEnum.AUTUMN.compareTo(SeasonEnum.AUTUMN);
System.out.println("compareTo = " + i);
//返回枚举类的类型
System.out.println("getDeclaringClass = " + SeasonEnum.AUTUMN.getDeclaringClass());
//如果指定的对象等于此枚举元素,则返回true。
System.out.println("equals = " + SeasonEnum.AUTUMN.equals("AUTUMN"));
}
结果:
autumn1 = AUTUMN
autumn2 = AUTUMN
values = [SPRING, SUMMER, AUTUMN, WINTER]
name1 = AUTUMN
name2 = AUTUMN
ordinal = 2
compareTo = 0
getDeclaringClass = class com.gongj.jsondate.controller.SeasonEnum
equals = false
枚举的使用
上面已经简单的介绍了枚举的定义与枚举的函数!那本节就带大家来看看在工作当中如何去使用枚举,哪些地方可以去使用枚举!
1、定义常量
就用上述的SeasonEnum
枚举类。
public static void main(String[] args) {
SeasonEnum type = SeasonEnum.AUTUMN;
if(SPRING.equals(type)){
System.out.println("春天:春暖花开");
}
if(SUMMER.equals(type)){
System.out.println("夏天:夏阳酷暑");
}
if(AUTUMN.equals(type)){
System.out.println("秋天:秋风习习");
}
if(WINTER.equals(type)){
System.out.println("冬天:冬日暖阳");
}
}
结果:秋天:秋风习习
在if
、switch
里的判断语句值都能用枚举进行替代,提高代码可读性。
2、参数接收
接口的请求参数值可以用枚举进行接收!比如OrderDTO
类的orderType
字段的类型,就可以使用枚举进行接收!那有什么好处呢?
- 1、代码可读性,会让其他开发者,一眼就知道订单类型有哪一些类型值。
- 2、明确订单类型的范围。可以防止用户随意传值。
public class OrderDTO {
private Long id;
private String orderName;
private SeasonEnum orderType;
}
这里还是用SeasonEnum
枚举类来演示。
提供对外接口
@PostMapping("/save")
public void save(@RequestBody OrderDTO req){
System.out.println( JSON.toJSONString(req));
}
然后进行调用:http://localhost:8080/save
,响应提示 value 不是声明的 Enum 实例名称之一
。
也就是说orderType
的值,只能为 SeasonEnum
枚举类所声明的实例。
3、码值转换
使用枚举类实现可以省略掉许多的 if/else
。大多数用于对接不同的系统,比如:接到一个与银行对接的功能,流程如下:前端 -》 本系统后端 -》调用银行接口。 其中有一个支付状态的码值。在自己系统 1-待支付,而在银行那边 0-待支付。两个系统之间的码值不一致,所以本系统就需要配置转换规则。而这时候就可以使用枚举类来进行实现。
3.1、编写枚举基类
编写枚举基类,所有枚举类都需要实现该接口,如果基类满足不了需求,子类可以随意扩展。
public interface BaseEnum {
String getKey();
String getValue();
String getDesc();
}
3.2、编写支付枚举类
实现BaseEnum
接口,并自行扩展 channel
、channelDesc
两个字段,并增加match
方法。
public enum PayEnum implements BaseEnum{
WAITING_PAY("1","0","待支付","unionpay","银联"),
SUCCESS_PAY("2","1","支付成功","unionpay","银联"),
FAIL_PAY("3","2","支付失败","unionpay","银联"),
ALIPAY_WAITING_PAY("1","3","待支付","alipay","支付宝");
private String key;
private String value;
private String desc;
//本类扩展字段 用于对接不同系统的支付状态
private String channel;
private String channelDesc;
private PayEnum(String key,String value,String desc,String channel,String channelDesc){
this.key = key;
this.value = value;
this.desc = desc;
this.channel = channel;
this.channelDesc = channelDesc;
}
@Override
public String getKey() {
return this.key;
}
@Override
public String getValue() {
return this.value;
}
@Override
public String getDesc() {
return this.desc;
}
public String getChannel() {
return channel;
}
public String getChannelDesc() {
return channelDesc;
}
/**
* 根据 key 和 channel 获得枚举实例
* @param key
* @param channel
* @return
*/
public static PayEnum match(String key, String channel) {
PayEnum[] enums = PayEnum.values();
for (PayEnum payEnum : enums) {
if (key.equals(payEnum.getKey()) && channel.equals(payEnum.getChannel()))
return payEnum;
}
return null;
}
}
3.3、测试
public static void main(String[] args) {
String key = "1";
String channel = "unionpay";
PayEnum match = match(key, channel);
System.out.println(match.getKey() + "==" + match.getValue()
+ "===" + match.getDesc() + "===" +
"==" + match.getChannel());
}
结果:1==0===待支付=====unionpay
在某些情况使用枚举,可以省略掉非常多的if/else
。
- 如你对本文有疑问或本文有错误之处,欢迎评论留言指出。如觉得本文对你有所帮助,欢迎点赞和关注。
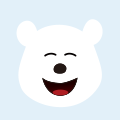